목차
반응형
안녕하세요. 훈츠 입니다. 이번 시간에는 구조체를 정의 해보려고 합니다.
구조체
- 개념 : 사용자가 정의 하는 데이터 형식
- 구성 : 필드 또는 멤버인 변수로 구성. 단, void 형은 사용할 수 없다.
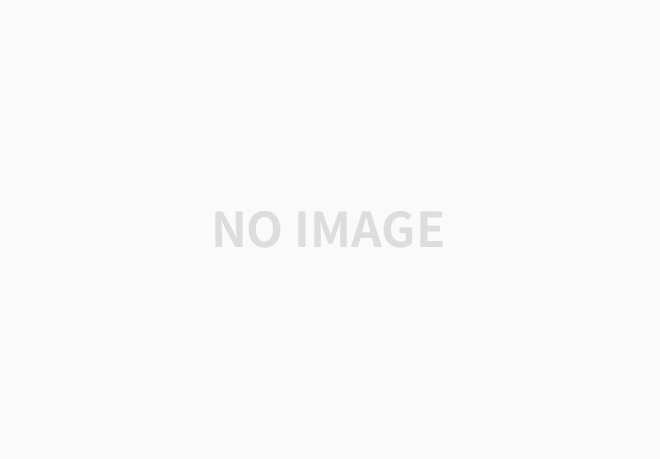
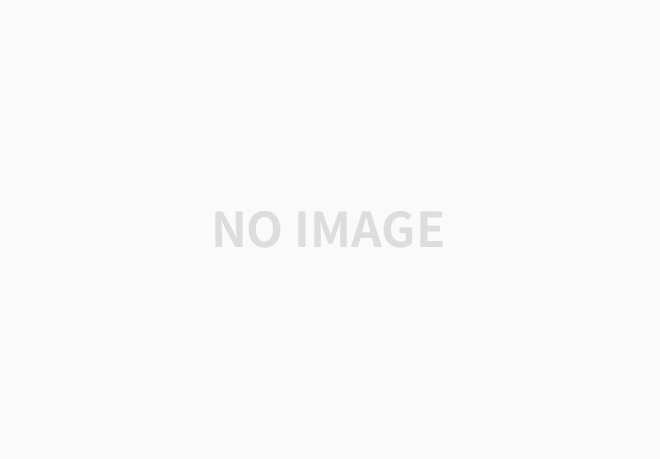
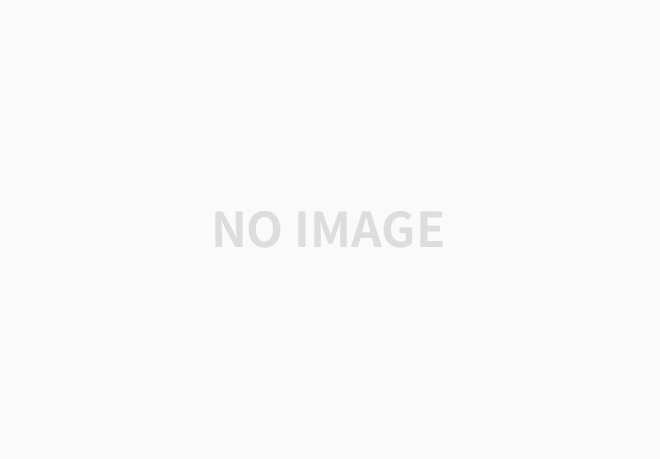
- 멤버 접근 연산자
- 변수 선언인 경우 ('.' dot 사용)
- Point.x = 10; Point.y = 20;
- 포인터 선언인 경우 ('->' 사용)
- pPoint->x = 10;, pPoint->y = 20;
- 다양한 구조체 변수 선언(일반변수와 동일하게 사용 및 선언)
- struct POINT Point;
- struct POINT *pPoint;
- struct POINT PointXY[3]; 등
#include <stdio.h>
#include <conio.h>
#include <memory.h>
#include <string.h>
#include <stdlib.h>
struct POINT {
int x;
int y;
};
int main() {
struct POINT p; //변수 선언
p.x = 10;
p.y = 20;
printf("%d %d\n", p.x, p.y);
struct POINT *pP,pp; //point 선언
pP = &pp;
pP->x = 30;
pP->y = 40;
printf("%d %d\n", pP->x, pP->y);
_getch();
return 0;
}
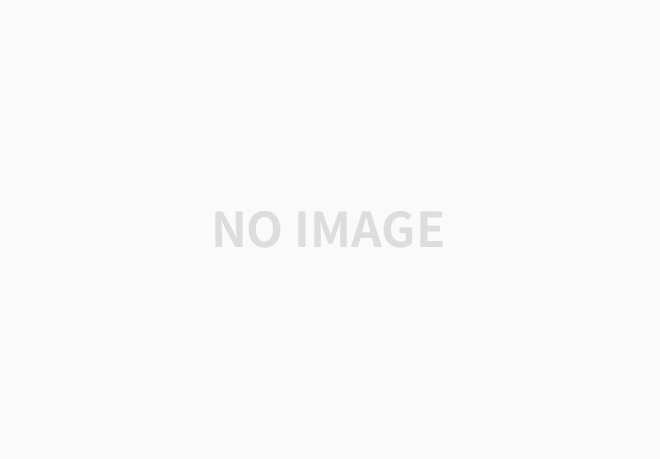
typedef
- 역활
- C 언어에서 정의된 기존 형식(int, char, ...)이나 사용자 정의 형식(struct)을 보다 짧게 또는 의미 있는 형식으로 재정의
- 형식
- typedef 기존 데이터형 새로운 데이터형;
- typedef int INT;
- typedef unsigned int UINT;
- typedef struct _POINT POINT;
- 긴문장을 짧게, 혹은 의미있게 바꿔서 사용
- struct 을 보다 짧게 또는 의미 있는 형식으로 재정의
#include <stdio.h>
#include <conio.h>
#include <memory.h>
#include <string.h>
#include <stdlib.h>
typedef struct _POINT {
int x;
int y;
}PT;
// typedef _POINT PT; //이렇게 선언도 가능합니다.
//함수로 구조체 받기 (값 복사)
int Cpoint(PT p) {
p.x = 50;
p.y = 60;
printf("Cpoint 함수 %d %d\n", p.x, p.y);
}
//함수로 구조체 포인터 받기 (값 포인터 접근)
int Cppoint(PT* p) {
p->x = 70;
p->y = 80;
printf("Cppoint 함수 %d %d\n", p->x, p->y);
}
int main() {
PT p; //변수 선언
p.x = 10;
p.y = 20;
printf("라인 1 %d %d\n", p.x, p.y);
PT *pP,pp; //point 선언
pP = &pp;
pP->x = 30;
pP->y = 40;
printf("라인 2 %d %d\n", pP->x, pP->y);
//함수(구조체) 호출
Cpoint(p);
printf("라인 3 %d %d\n", p.x, p.y);
//함수(포인터) 호출
Cppoint(pP);
printf("라인 4 %d %d\n", pP->x, pP->y);
_getch();
return 0;
}
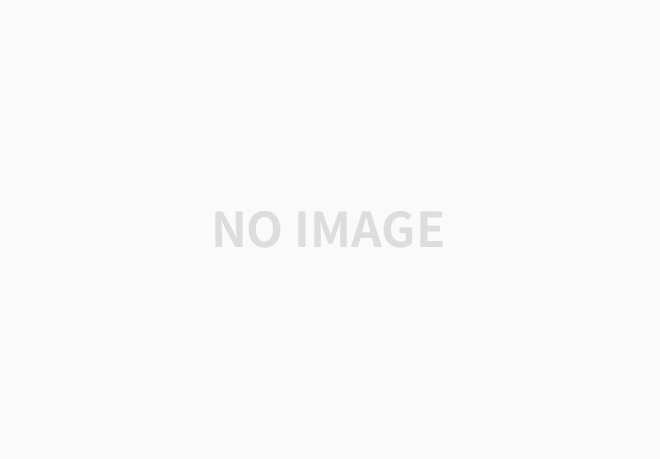
정리
- 구조체는 데이터형을 사용자 임의로 재정의 하는 것입니다.
- typedef 을 통해 데이터형을 간단히 할 수 있습니다.
'컴퓨터 언어 > C' 카테고리의 다른 글
[C] C언어 8일차 기타 문법2 (지역, 전역, 정적 변수 사용 범위) (0) | 2020.03.17 |
---|---|
[C] C언어 8일차 기타 문법1 (enum, goto, label, union, 전처리기 지시문) (0) | 2020.03.17 |
[C] C언어 7일차 포인터2 (0) | 2020.03.16 |
[C] C언어 6일차 포인터 (0) | 2020.03.15 |
[C] C언어 5일차 배열 (0) | 2020.03.14 |